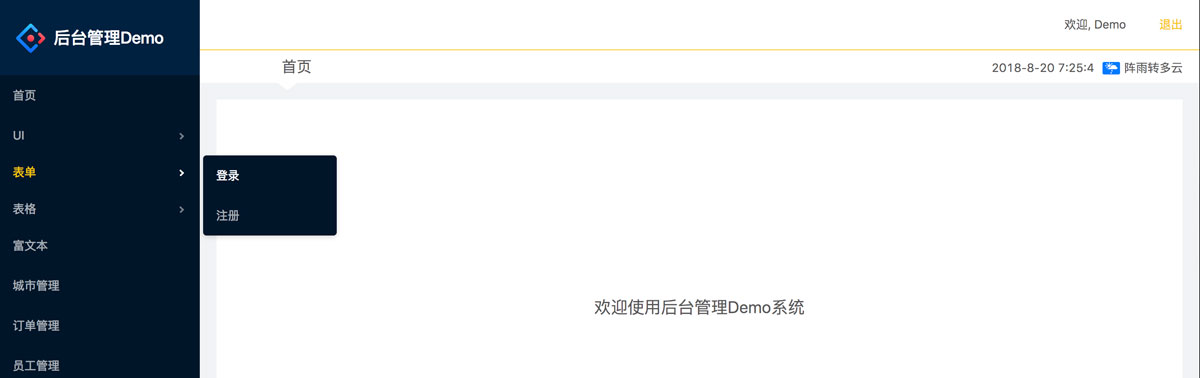
基础知识
React 特点
- Declarative 声明式编码
- Component-Based 组件化编码
- 高效-高效的 DOM Diff算法,最小化页面重绘
- 单向数据流
Vue & React 生态
Vue + Vue-Router + Vuex + Axios + Babel + Webpack
React + React-Router – Redux + Axios + Babel +Webpack
React 脚手架、Yarn
1 2 3 4 5 6 7 8 9 10 11 |
# npm sudo npm install -g create-react-app create-react-app my-app cd my-app npm start # yarn(sudo npm -g install yarn) yarn init yarn add yarn remove yarn / yarn install # 进入项目目录 yarn start 即可启动项目 |
React 生命周期
getDefaultProps
getInitialState
componentWillMount
render
componentDidMount
componentWillReceiveProps
shouldComponentUpdate
componentWillUpdate
componentDidUpdate
componentWillUnmount
1 2 3 4 5 6 7 8 9 10 11 |
handleAdd=()=>{ ... } handleClick(){ ... } # 调用方法 <button onClick={this.handleAdd}>点击一下</button> <button onClick={this.handleClick.bind(this)}>点击一下</button |
AntD 基于LESS,可暴露 WebPack 来进行相应修改
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 |
# 安装基础插件 yarn add react-router-dom axios less-loader less@^2.7.3 jsonp # 暴露webpack 文件 yarn eject # config/webpack.config.dev.js, config/webpack.config.prod.js # 拷贝test: /\.less$/代码块以支持 LESS,以及设置主题色 { test: /\.less$/, .... { loader:require.resolve('less-loader'), options: { modules: false, modifyVars: { "@primary-color": "#f9c700" } } } ], }, # 修改后重启项目 # 安装 antd yarn add antd # 实现按需加载配置,这样无需再每次导入 antd 的 css 文件 yarn add babel-plugin-import # config/webpack.config.dev.js, config/webpack.config.prod.js { test: /\.(js|jsx|mjs)$/, ... options: { plugins:[ ['import', [{ libraryName:'antd', style:true }]] ], ... }, }, # 新版中无法使用前述方法,按需加载及修改主题方法如下: { test: /\.less$/, use: [{ loader: 'style-loader', }, { loader: 'css-loader', // translates CSS into CommonJS }, { loader: 'less-loader', // compiles Less to CSS options: { modifyVars: {//修改后的主题色 '@primary-color': '#f9c700', }, javascriptEnabled: true, }, }], }, ... { test: /\.(js|mjs|jsx|ts|tsx)$/, ... plugins: [ ... [require.resolve('babel-plugin-import'), { libraryName: 'antd', style: true }] ], ... }, |
React Router 4.0
react-router(基础包)
react-router-dom(针对浏览器端,已包含 react-router)提供了BrowserRouter, HashRouter, Route(path, exact, component, render), Link, NavLink, switch, redirect
yarn add react-router-dom
获取动态路由参数:this.props.match.params.xxx
React 文件的基本写法:
1 2 3 4 5 6 7 8 9 10 |
import React from 'react' export default class Header extends React.Component{ render(){ return( <div>Header</div> ); } } |
获取子节点:this.props.children
Mock 数据:https://www.easy-mock.com
常见问题
1、A template was not provided. This is likely because you’re using an outdated version of create-react
1 2 |
npm uninstall -g create-react-app npx create-react-app frontend |